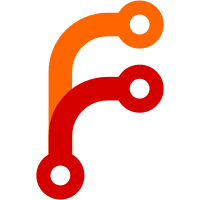
* wip * wip * wip * Update following.ts * wip * wip * wip * Update resolve-user.ts * maxQueryExecutionTime * wip * wip
132 lines
2.8 KiB
TypeScript
132 lines
2.8 KiB
TypeScript
import { Users, Followings } from '@/models/index.js';
|
|
import { ILocalUser, IRemoteUser, User } from '@/models/entities/user.js';
|
|
import { deliver } from '@/queue/index.js';
|
|
|
|
//#region types
|
|
interface IRecipe {
|
|
type: string;
|
|
}
|
|
|
|
interface IFollowersRecipe extends IRecipe {
|
|
type: 'Followers';
|
|
}
|
|
|
|
interface IDirectRecipe extends IRecipe {
|
|
type: 'Direct';
|
|
to: IRemoteUser;
|
|
}
|
|
|
|
const isFollowers = (recipe: any): recipe is IFollowersRecipe =>
|
|
recipe.type === 'Followers';
|
|
|
|
const isDirect = (recipe: any): recipe is IDirectRecipe =>
|
|
recipe.type === 'Direct';
|
|
//#endregion
|
|
|
|
export default class DeliverManager {
|
|
private actor: { id: User['id']; host: null; };
|
|
private activity: any;
|
|
private recipes: IRecipe[] = [];
|
|
|
|
/**
|
|
* Constructor
|
|
* @param actor Actor
|
|
* @param activity Activity to deliver
|
|
*/
|
|
constructor(actor: { id: User['id']; host: null; }, activity: any) {
|
|
this.actor = actor;
|
|
this.activity = activity;
|
|
}
|
|
|
|
/**
|
|
* Add recipe for followers deliver
|
|
*/
|
|
public addFollowersRecipe() {
|
|
const deliver = {
|
|
type: 'Followers',
|
|
} as IFollowersRecipe;
|
|
|
|
this.addRecipe(deliver);
|
|
}
|
|
|
|
/**
|
|
* Add recipe for direct deliver
|
|
* @param to To
|
|
*/
|
|
public addDirectRecipe(to: IRemoteUser) {
|
|
const recipe = {
|
|
type: 'Direct',
|
|
to,
|
|
} as IDirectRecipe;
|
|
|
|
this.addRecipe(recipe);
|
|
}
|
|
|
|
/**
|
|
* Add recipe
|
|
* @param recipe Recipe
|
|
*/
|
|
public addRecipe(recipe: IRecipe) {
|
|
this.recipes.push(recipe);
|
|
}
|
|
|
|
/**
|
|
* Execute delivers
|
|
*/
|
|
public async execute() {
|
|
if (!Users.isLocalUser(this.actor)) return;
|
|
|
|
const inboxes = new Set<string>();
|
|
|
|
// build inbox list
|
|
for (const recipe of this.recipes) {
|
|
if (isFollowers(recipe)) {
|
|
// followers deliver
|
|
const followers = await Followings.findBy({
|
|
followeeId: this.actor.id,
|
|
});
|
|
|
|
for (const following of followers) {
|
|
if (Followings.isRemoteFollower(following)) {
|
|
const inbox = following.followerSharedInbox || following.followerInbox;
|
|
inboxes.add(inbox);
|
|
}
|
|
}
|
|
} else if (isDirect(recipe)) {
|
|
// direct deliver
|
|
const inbox = recipe.to.inbox;
|
|
if (inbox) inboxes.add(inbox);
|
|
}
|
|
}
|
|
|
|
// deliver
|
|
for (const inbox of inboxes) {
|
|
deliver(this.actor, this.activity, inbox);
|
|
}
|
|
}
|
|
}
|
|
|
|
//#region Utilities
|
|
/**
|
|
* Deliver activity to followers
|
|
* @param activity Activity
|
|
* @param from Followee
|
|
*/
|
|
export async function deliverToFollowers(actor: { id: ILocalUser['id']; host: null; }, activity: any) {
|
|
const manager = new DeliverManager(actor, activity);
|
|
manager.addFollowersRecipe();
|
|
await manager.execute();
|
|
}
|
|
|
|
/**
|
|
* Deliver activity to user
|
|
* @param activity Activity
|
|
* @param to Target user
|
|
*/
|
|
export async function deliverToUser(actor: { id: ILocalUser['id']; host: null; }, activity: any, to: IRemoteUser) {
|
|
const manager = new DeliverManager(actor, activity);
|
|
manager.addDirectRecipe(to);
|
|
await manager.execute();
|
|
}
|
|
//#endregion
|