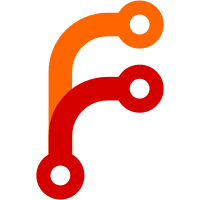
* refactor(sw): remove dead code * refactor(sw): remove dead code * refactor(sw): remove dead code * refactor(sw): remove dead code * refactor(sw): remove dead code * refactor(sw): remove dead code * refactor(sw): 冗長な部分を変更 * refactor(sw): 使われていない煩雑な機能を削除 * refactor(sw): remove dead code * refactor(sw): URL文字列の作成に`URL`を使うように * refactor(sw): 型アサーションの削除とそれに伴い露呈したエラーへの対処 * refactor(sw): `append` -> `set` in `URLSearchParams` * refactor(sw): `any`の削除とそれに伴い露呈したエラーへの対処 * refactor(sw): 型アサーションの削除とそれに伴い露呈したエラーへの対処 対処と言っても`throw`するだけ。いままでもこの状況ではエラーが投げられていたはずなので、この対処により新たな問題が起きることはないはず。 * refactor(sw): i18n loading * refactor(sw): 型推論がうまくできる書き方に変更 `codes`が`(string | undefined)[]`から`string[]`になった * refactor(sw): クエリ文字列の作成に`URLSearchParams`を使うように * refactor(sw): `findClient` * refactor(sw): `openClient`における`any`や`as`の書き換え * refactor(sw): `openPost`における`any`の書き換え * refactor(sw): `let` -> `const` * refactor(sw): `any` -> `unknown` * cleanup(sw): import * cleanup(sw) * cleanup(sw): `?.` * cleanup(sw/.eslintrc.js) * refactor(sw): `@typescript-eslint/explicit-function-return-type` * refactor(sw): `@typescript-eslint/no-unused-vars` * refactor(sw): どうしようもないところに`eslint-disable-next-line`を * refactor(sw): `import/no-default-export` * update operations.ts * throw new Error --------- Co-authored-by: tamaina <tamaina@hotmail.co.jp> Co-authored-by: Kainoa kanter <kainoa@t1c.dev>
121 lines
3.1 KiB
TypeScript
121 lines
3.1 KiB
TypeScript
/*
|
||
* Operations
|
||
* 各種操作
|
||
*/
|
||
import * as Misskey from "calckey-js";
|
||
import type { SwMessage, SwMessageOrderType } from "@/types";
|
||
import { getAccountFromId } from "@/scripts/get-account-from-id";
|
||
import { getUrlWithLoginId } from "@/scripts/login-id";
|
||
|
||
export const cli = new Misskey.api.APIClient({
|
||
origin,
|
||
fetch: (...args): Promise<Response> => fetch(...args),
|
||
});
|
||
|
||
export async function api<
|
||
E extends keyof Misskey.Endpoints,
|
||
O extends Misskey.Endpoints[E]["req"],
|
||
>(
|
||
endpoint: E,
|
||
userId?: string,
|
||
options?: O,
|
||
): Promise<void | ReturnType<typeof cli.request<E, O>>> {
|
||
let account: { token: string; id: string } | void;
|
||
|
||
if (userId) {
|
||
account = await getAccountFromId(userId);
|
||
if (!account) return;
|
||
}
|
||
|
||
return cli.request(endpoint, options, account?.token);
|
||
}
|
||
|
||
// mark-all-as-read送出を1秒間隔に制限する
|
||
const readBlockingStatus = new Map<string, boolean>();
|
||
export function sendMarkAllAsRead(
|
||
userId: string,
|
||
): Promise<null | undefined | void> {
|
||
if (readBlockingStatus.get(userId)) return Promise.resolve();
|
||
readBlockingStatus.set(userId, true);
|
||
return new Promise((resolve) => {
|
||
setTimeout(() => {
|
||
readBlockingStatus.set(userId, false);
|
||
api("notifications/mark-all-as-read", userId).then(resolve, resolve);
|
||
}, 1000);
|
||
});
|
||
}
|
||
|
||
// rendered acctからユーザーを開く
|
||
export function openUser(
|
||
acct: string,
|
||
loginId?: string,
|
||
): ReturnType<typeof openClient> {
|
||
return openClient("push", `/@${acct}`, loginId, { acct });
|
||
}
|
||
|
||
// noteIdからノートを開く
|
||
export function openNote(
|
||
noteId: string,
|
||
loginId?: string,
|
||
): ReturnType<typeof openClient> {
|
||
return openClient("push", `/notes/${noteId}`, loginId, { noteId });
|
||
}
|
||
|
||
// noteIdからノートを開く
|
||
export function openAntenna(
|
||
antennaId: string,
|
||
loginId: string,
|
||
): ReturnType<typeof openClient> {
|
||
return openClient("push", `/timeline/antenna/${antennaId}`, loginId, {
|
||
antennaId,
|
||
});
|
||
}
|
||
|
||
// post-formのオプションから投稿フォームを開く
|
||
export async function openPost(
|
||
options: {
|
||
initialText?: string;
|
||
reply?: Misskey.entities.Note;
|
||
renote?: Misskey.entities.Note;
|
||
},
|
||
loginId?: string,
|
||
): ReturnType<typeof openClient> {
|
||
// クエリを作成しておく
|
||
const url = "/share";
|
||
const query = new URLSearchParams();
|
||
if (options.initialText) query.set("text", options.initialText);
|
||
if (options.reply) query.set("replyId", options.reply.id);
|
||
if (options.renote) query.set("renoteId", options.renote.id);
|
||
|
||
return openClient("post", `${url}?${query}`, loginId, { options });
|
||
}
|
||
|
||
export async function openClient(
|
||
order: SwMessageOrderType,
|
||
url: string,
|
||
loginId?: string,
|
||
query: Record<string, SwMessage[string]> = {},
|
||
): Promise<WindowClient | null> {
|
||
const client = await findClient();
|
||
|
||
if (client) {
|
||
client.postMessage({
|
||
type: "order",
|
||
...query,
|
||
order,
|
||
loginId,
|
||
url,
|
||
} satisfies SwMessage);
|
||
return client;
|
||
}
|
||
|
||
return self.clients.openWindow(getUrlWithLoginId(url, loginId!));
|
||
}
|
||
|
||
export async function findClient(): Promise<WindowClient | null> {
|
||
const clients = await globalThis.clients.matchAll({
|
||
type: "window",
|
||
});
|
||
return clients.find((c) => !new URL(c.url).searchParams.has("zen")) ?? null;
|
||
}
|