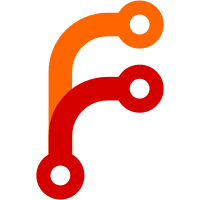
Properties can now have values of different types, and they are registered with their name, either to a layout class or globally. Layout classes are also registered with their name.
130 lines
4.3 KiB
C
130 lines
4.3 KiB
C
#include "clay.h"
|
|
#include "layout.h"
|
|
|
|
static struct clay_layout_class_s layout_class_text = {};
|
|
|
|
|
|
static clay_prop_definition_in prop_align = {
|
|
.keywords = CLAY_PROP_DEF_KEYWORDS(
|
|
CLAY_PROP_DEF_KEYWORD("left", 0),
|
|
CLAY_PROP_DEF_KEYWORD("center", 1),
|
|
CLAY_PROP_DEF_KEYWORD("right", 2),
|
|
CLAY_PROP_DEF_KEYWORD("justify", 3),
|
|
),
|
|
};
|
|
|
|
static clay_prop_definition_in prop_vertical_align = {
|
|
.keywords = CLAY_PROP_DEF_KEYWORDS(
|
|
CLAY_PROP_DEF_KEYWORD("top", 0),
|
|
CLAY_PROP_DEF_KEYWORD("middle", 1),
|
|
CLAY_PROP_DEF_KEYWORD("bottom", 2),
|
|
),
|
|
};
|
|
|
|
static clay_prop_definition_in prop_font_size = {
|
|
.allow_int = true,
|
|
.allow_float = true,
|
|
.allow_units = CLAY_PROP_UNIT_PT | CLAY_PROP_UNIT_NONE,
|
|
.inherits = true,
|
|
};
|
|
|
|
static clay_prop_definition_in prop_line_height = {
|
|
.allow_int = true,
|
|
.allow_float = true,
|
|
.allow_units = CLAY_PROP_UNIT_PT | CLAY_PROP_UNIT_NONE,
|
|
.keywords = CLAY_PROP_DEF_KEYWORDS(CLAY_PROP_DEF_KEYWORD("auto", 1)),
|
|
.inherits = true,
|
|
};
|
|
|
|
|
|
static clay_prop_definition_in prop_font_weight = {
|
|
.allow_int = true,
|
|
.allow_units = CLAY_PROP_UNIT_NONE,
|
|
.keywords = CLAY_PROP_DEF_KEYWORDS(
|
|
CLAY_PROP_DEF_KEYWORD("thin", 100),
|
|
CLAY_PROP_DEF_KEYWORD("extra-light", 200),
|
|
CLAY_PROP_DEF_KEYWORD("ultra-light", 200),
|
|
CLAY_PROP_DEF_KEYWORD("light", 300),
|
|
CLAY_PROP_DEF_KEYWORD("normal", 400),
|
|
CLAY_PROP_DEF_KEYWORD("regular", 400),
|
|
CLAY_PROP_DEF_KEYWORD("medium", 500),
|
|
CLAY_PROP_DEF_KEYWORD("semi-bold", 600),
|
|
CLAY_PROP_DEF_KEYWORD("demi-bold", 600),
|
|
CLAY_PROP_DEF_KEYWORD("bold", 700),
|
|
CLAY_PROP_DEF_KEYWORD("extra-bold", 800),
|
|
CLAY_PROP_DEF_KEYWORD("black", 900),
|
|
),
|
|
.inherits = true,
|
|
};
|
|
|
|
|
|
enum text_font_style {
|
|
FONT_STYLE_NORMAL,
|
|
FONT_STYLE_ITALIC,
|
|
};
|
|
|
|
static clay_prop_definition_in prop_font_style = {
|
|
.allow_int = true,
|
|
.allow_units = CLAY_PROP_UNIT_NONE,
|
|
.keywords = CLAY_PROP_DEF_KEYWORDS(
|
|
CLAY_PROP_DEF_KEYWORD("normal", FONT_STYLE_NORMAL),
|
|
CLAY_PROP_DEF_KEYWORD("italic", FONT_STYLE_ITALIC),
|
|
),
|
|
.inherits = true,
|
|
};
|
|
|
|
static clay_prop_definition_in prop_language = {
|
|
.keywords = CLAY_PROP_DEF_KEYWORDS(
|
|
CLAY_PROP_DEF_KEYWORD("revert", 1),
|
|
),
|
|
.allow_string = true,
|
|
.inherits = true,
|
|
};
|
|
|
|
enum text_font_family {
|
|
FONT_FAMILY_SERIF,
|
|
FONT_FAMILY_SANS_SERIF,
|
|
FONT_FAMILY_MONOSPACE,
|
|
FONT_FAMILY_EMOJI,
|
|
FONT_FAMILY_REVERT,
|
|
};
|
|
|
|
static clay_prop_definition_in prop_font_family = {
|
|
.keywords = CLAY_PROP_DEF_KEYWORDS(
|
|
CLAY_PROP_DEF_KEYWORD("serif", FONT_FAMILY_SERIF),
|
|
CLAY_PROP_DEF_KEYWORD("sans-serif", FONT_FAMILY_SANS_SERIF),
|
|
CLAY_PROP_DEF_KEYWORD("monospace", FONT_FAMILY_MONOSPACE),
|
|
CLAY_PROP_DEF_KEYWORD("emoji", FONT_FAMILY_EMOJI),
|
|
CLAY_PROP_DEF_KEYWORD("revert", FONT_FAMILY_REVERT),
|
|
),
|
|
.allow_string = true,
|
|
.inherits = true,
|
|
};
|
|
|
|
|
|
static clay_prop_definition_in prop_text_color = {
|
|
.allow_color = true,
|
|
.inherits = true,
|
|
};
|
|
|
|
|
|
static clay_prop_definition_in prop_text_text = {
|
|
.allow_string = true,
|
|
};
|
|
|
|
|
|
void clay_text_register(clay_ctx ctx) {
|
|
clay_ctx_register_layout_class(ctx, "text", &layout_class_text);
|
|
|
|
clay_ctx_register_class_property(ctx, "text", "align", &prop_align);
|
|
clay_ctx_register_class_property(ctx, "text", "vertical-align", &prop_vertical_align);
|
|
clay_ctx_register_class_property(ctx, "text", "text", &prop_text_text);
|
|
|
|
clay_ctx_register_global_property(ctx, "font-size", &prop_font_size);
|
|
clay_ctx_register_global_property(ctx, "font-weight", &prop_font_weight);
|
|
clay_ctx_register_global_property(ctx, "font-family", &prop_font_family);
|
|
clay_ctx_register_global_property(ctx, "font-style", &prop_font_style);
|
|
clay_ctx_register_global_property(ctx, "line-height", &prop_line_height);
|
|
clay_ctx_register_global_property(ctx, "language", &prop_language);
|
|
clay_ctx_register_global_property(ctx, "text-color", &prop_text_color);
|
|
} |