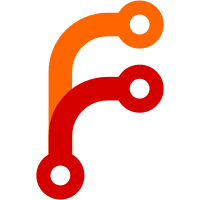
the typechecker project can collect all the top level types from a file, which is pretty cool I think (except for pointers, those aren't implemented yet...)
61 lines
2.2 KiB
C#
61 lines
2.2 KiB
C#
using meowlang.parser.antlr;
|
|
|
|
namespace meowlang.parser;
|
|
|
|
public class TypeReferenceVisitorNya : MeowBaseVisitorNya<TypeReferenceModel>
|
|
{
|
|
public override TypeReferenceModel VisitTypeReference(MeowParser.TypeReferenceContext context)
|
|
{
|
|
var type = Visit(context.nonPointerTypeReference());
|
|
if (context.pointer != null)
|
|
{
|
|
return new PointerTypeReferenceModel(context.GetSpan(), type);
|
|
}
|
|
|
|
return type;
|
|
}
|
|
|
|
public override TypeReferenceModel VisitTypeName(MeowParser.TypeNameContext context)
|
|
{
|
|
var name = new IdentifierNameVisitorNya().Visit(context.identifierName());
|
|
var typeParameters = context._genericType.Select(Visit).ToList();
|
|
var nestedName = context._name.Select(x => x.Text).ToList();
|
|
return new TypeNameModel(context.GetSpan(), name, typeParameters, nestedName);
|
|
}
|
|
|
|
public override TypeReferenceModel VisitArrayType(MeowParser.ArrayTypeContext context)
|
|
{
|
|
var type = Visit(context.typeReference());
|
|
if (context.length == null)
|
|
{
|
|
return new SliceTypeModel(context.GetSpan(), type);
|
|
}
|
|
|
|
if (context.length.Text.Contains('.') || context.length.Text.Contains('-'))
|
|
{
|
|
throw new InvalidArrayLengthException(context.length);
|
|
}
|
|
|
|
var size = uint.Parse(context.length.Text.Replace("_", ""));
|
|
|
|
return new ArrayTypeModel(context.GetSpan(), type, size);
|
|
}
|
|
|
|
public override TypeReferenceModel VisitTupleType(MeowParser.TupleTypeContext context)
|
|
{
|
|
var types = context.typeReference().Select(Visit).ToList();
|
|
return new TupleTypeModel(context.GetSpan(), types);
|
|
}
|
|
|
|
public override TypeReferenceModel VisitStructType(MeowParser.StructTypeContext context)
|
|
{
|
|
var members = context.structMember().Select(x => new StructMemberVisitorNya().Visit(x)).ToList();
|
|
return new StructTypeModel(context.GetSpan(), members);
|
|
}
|
|
|
|
public override TypeReferenceModel VisitEnumType(MeowParser.EnumTypeContext context)
|
|
{
|
|
var members = context.enumMember().Select(x => new EnumMemberVisitorNya().Visit(x)).ToList();
|
|
return new EnumTypeModel(context.GetSpan(), members);
|
|
}
|
|
} |