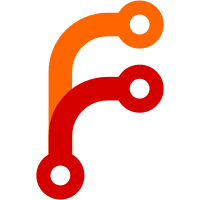
the typechecker project can collect all the top level types from a file, which is pretty cool I think (except for pointers, those aren't implemented yet...)
45 lines
1.4 KiB
C#
45 lines
1.4 KiB
C#
namespace meowlang.typechecker;
|
|
|
|
public abstract record GenericTypeDescription : TypeDescription
|
|
{
|
|
protected readonly List<string> GenericNames;
|
|
|
|
public int Arity => GenericNames.Count;
|
|
|
|
public GenericTypeDescription(List<string> genericNames)
|
|
{
|
|
GenericNames = genericNames;
|
|
}
|
|
|
|
protected void CheckGenericNames(List<GenericTypeId> genericTypes)
|
|
{
|
|
foreach (var typeId in genericTypes)
|
|
{
|
|
if (typeId.Key.IsGeneric)
|
|
{
|
|
if (GenericNames.IndexOf(typeId.Key.GenericParameterName!) < 0)
|
|
{
|
|
throw new UndeclaredGenericParameterException(typeId.Key.GenericParameterName!);
|
|
}
|
|
}
|
|
|
|
CheckGenericNames(typeId.GenericParams);
|
|
}
|
|
}
|
|
public abstract TypeDescription Concretize(List<Guid> typeParams);
|
|
|
|
|
|
|
|
protected TypeId ConcretizeGenericType(GenericTypeId genericType, List<Guid> concreteTypes)
|
|
{
|
|
return new TypeId(ConcretizeGenericTypeRef(genericType.Key, concreteTypes),
|
|
genericType.GenericParams.Select(x => ConcretizeGenericType(x, concreteTypes)).ToList());
|
|
}
|
|
|
|
private Guid ConcretizeGenericTypeRef(PossiblyGenericTypeRef typeRef, List<Guid> concreteTypes)
|
|
{
|
|
if (!typeRef.IsGeneric) return typeRef.TypeKey!.Value;
|
|
var typeIndex = GenericNames.IndexOf(typeRef.GenericParameterName!);
|
|
return concreteTypes[typeIndex];
|
|
}
|
|
} |